Last Updated | | Ratings | | Unique User Downloads | | Download Rankings |
2020-02-17 (Less than 1 hour ago)  | | Not enough user ratings | | Total: 301 | | All time: 7,266 This week: 324 |
|
Description | | Author |
This package can generate complete thoughts from a verb and a noun.
It takes a verb and an noun and uses the Moby thesaurus file to extract synonyms.
Complete thoughts may be found by looking for any of the verb synonyms in the noun synonyms. Innovation Award
 January 2017
Number 18
Prize: PHP Tools for Visual Studio Personal license |
Well formed sentences are based on grammatically correct combination of verbs and nouns, thus forming what is called complete thoughts.
This package provides a solution for generating complete thoughts from verbs and nouns.
It also allows verifying if sentences may have been written by bots that generate fake text by checking if the combination of verb and noun words is present in sentences.
Manuel Lemos |
| |
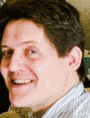 |
|
Innovation award
 Nominee: 2x |
|
Details
PHP Moby NLP

Gets the "complete thought" from a verb and a noun using Moby Thesaurus
What does it do?
- It uses the Moby Thesaurus to get the "complete thought" from a verb and a noun.
- This is meant for Natural Language Processing (NLP) tasks.
How does it work?
- The code looks for "complete thoughts", see the link to Wikipedia here:
`
https://simple.wikipedia.org/wiki/Simple_sentence
`
- First: open the index.php, a form will appear.
- Second: enter a verb, like "walk", "talk" or "run". It is best to user the base form or lemma. So do not use "running", but use "run". This program can be used to get the lemma for you:
`
https://github.com/DennisDeSwart/php-stanford-corenlp-adapter
https://www.phpclasses.org/package/10056-PHP-Natural-language-processing-using-Stanford-server.html
`
- Third, enter a noun like "dog", "cat", "house" or "car".
- Finally, press Submit, wait for the result. The result may take up to 2 seconds.
What can I do with the result?
- First, you can use it in spam killers: if there are complete thoughts in a text, the text is probably not fake.
- Second: you can use this for creating reports and understanding text.
- Third: this could be used as suggestions in search engines.
Requirements
- PHP 5.3 or higher: it also works on PHP 7
Installation using Composer
You can install the adapter by putting the following line into your composer.json and running a composer update
{
"require": {
"dennis-de-swart/php-moby-nlp": "*"
}
}
Recommended practices
- Looking up words in the thesaurus can take up to 2 seconds. You should only lookup words if you need to.
- To select the most important words like verbs and nouns, you can use a NLP parser like Stanford's CoreNLP
- To use Stanford CoreNLP check these links:
`
https://github.com/DennisDeSwart/php-stanford-corenlp-adapter
http://stanfordnlp.github.io/CoreNLP/corenlp-server.html
`
Can I use a different set of words (=thesaurus)?
Yes. This script uses the Moby Thesaurus that is based on the English language from 1996. It doesn't have many modern words. You can change the Thesaurus by using an API.
However, this is not programmed yet. To make it happen, there needs to be an API connection. Also the existing Synonym function needs to be re-written to use this API.
I want to write a script to use the Urban Dictionary API. This would make it talk "urban". Here is an example on how to connect:
https://github.com/zdict/zdict/wiki/Urban-dictionary-API-documentation
Example output
See "example_write_document.PNG"
Any questions?
Please let me know.
Credits
Brent Rossen, orginal author of the MobyThesaurus.php class
https://github.com/phyous/moby-thesaurus
|
Applications that use this package |
|
No pages of applications that use this class were specified.
If you know an application of this package, send a message to the author to add a link here.